Gilp: A node tool to implement pre-commit and other git hooks
Pablo Ricco
November 15, 2016
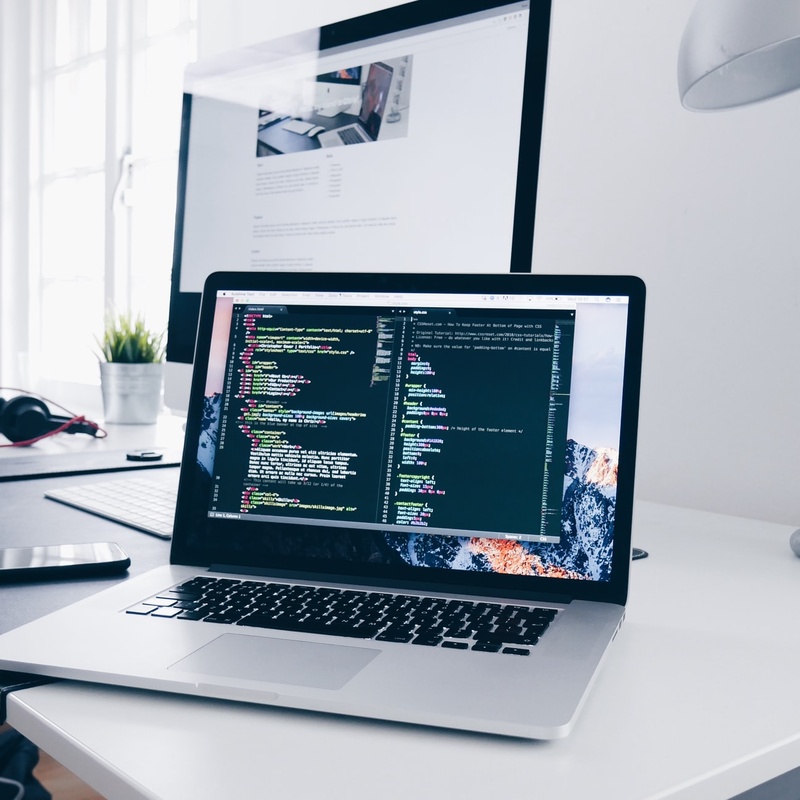
Git has a lot of hooks for client and server side but the most used and known hook is pre-commit, where you can run some validations (like linters) and cancel a commit if something fails. Gilp is a tool to define (using gulp tasks), install and run hooks.
Why gilp over gulp?
There are 2 main reasons:
-
The regular pre-commit hook managers run using the local file's content instead of git staged content. Gulp comes with
vinyl
, a virtual file format where we can create an in-memory version of a file to use as content for the tasks. Likegulp.src
, we created a new stream provider for that:srcFromStaged
. If we need to run the same tools but over acommit
,branch
ortag
instead (e.g. in a CI), you can do it usingsrcFromCommit
without acheckout
. -
Gulp has plenty of plugins ready to use, check here.
Yarn, please.
We recommend to use yarn
instead of npm
because the error report is less verbose when gulp
returns a non-zero code (on error) so we can focus on the "real" error.
Installation
yarn add --dev gilp
Usage
You can use any gulp or gilp plugin.
How to define a hook
var gulp = require('gulp');
var eslint = require('gulp-eslint');
var gilp = require('gilp')(gulp);
gilp.hook('pre-commit', function () {
return gilp.srcFromStaged(['**/*.js'])
.pipe(eslint())
.pipe(eslint.failAfterError());
});
** Get a stream of files to be committed (staged content):**
gilp.srcFromStaged();
** Get a stream of files from a commit:**
gilp.srcFromCommit('e3bca34');
Install defined hooks (.git/hooks)
Gilp auto define a gulp task to install the git hooks properly because each hook should be defined under the .git/hooks
folder.
yarn run gulp gilp-install
To auto-install the hooks after yarn [install]
, add in your package.json
the
following postinstall
command:
{
// ...
"scripts": {
// ...
"gulp": "gulp",
"postinstall": "gulp gilp-install"
},
// ...
}
Run a task on the CI to check the commit
var gulp = require('gulp');
var eslint = require('gulp-eslint');
var gilp = require('gilp')(gulp);
gulp.task('check-commit', function () {
return gilp.srcFromCommit('e3bca34', ['**/*.js'])
.pipe(eslint())
.pipe(eslint.failAfterError());
});
Final notes
This is a open source project under MIT license and can be found in our github organization: https://github.com/sophilabs/gilp
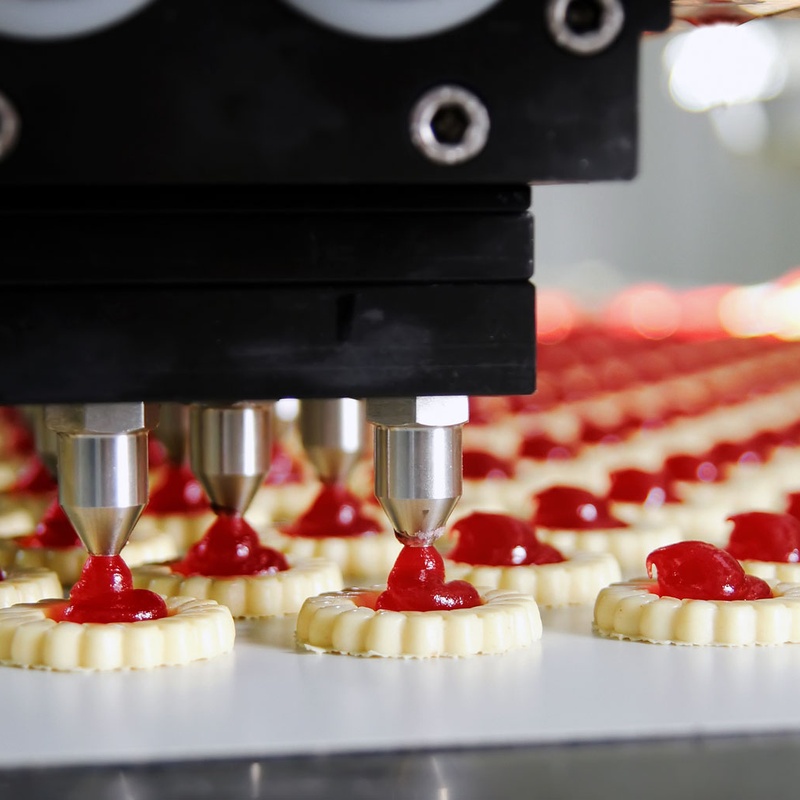
Code quality enforcement tools
This is a series of posts describing some of the tools we use to keep our code clean and enforce best practices and project-specific standards. Read on, to find out more.
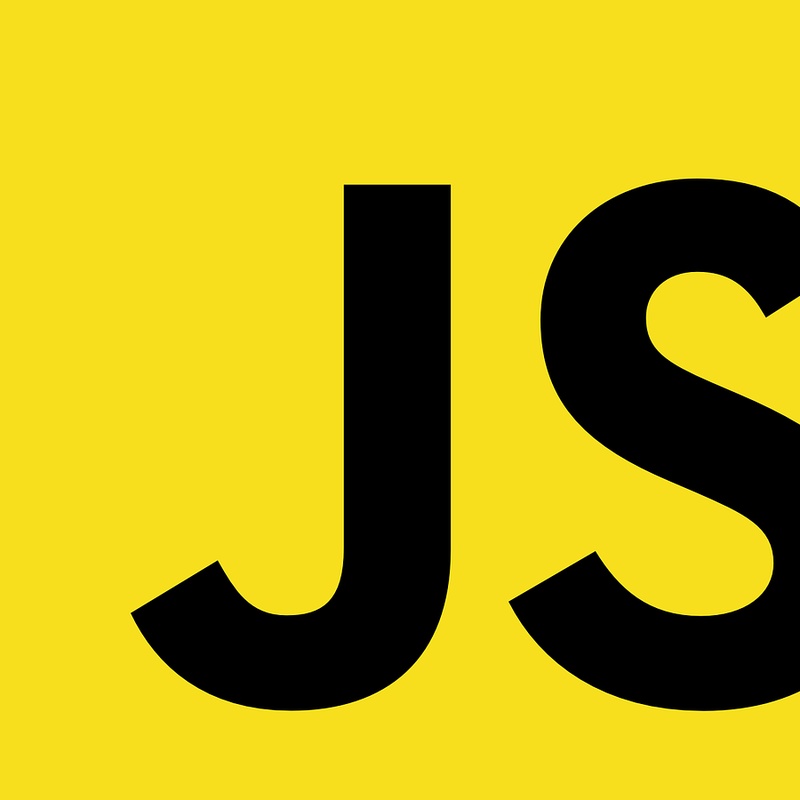
The Javascript Meetups arrived in Uruguay
The organizer of the Meetup was Pablo Ricco, Sophilabs and Universidad Católica were the official sponsors of the event. As in every meetup, help from any other volunteer and sponsors is highly appreciated.
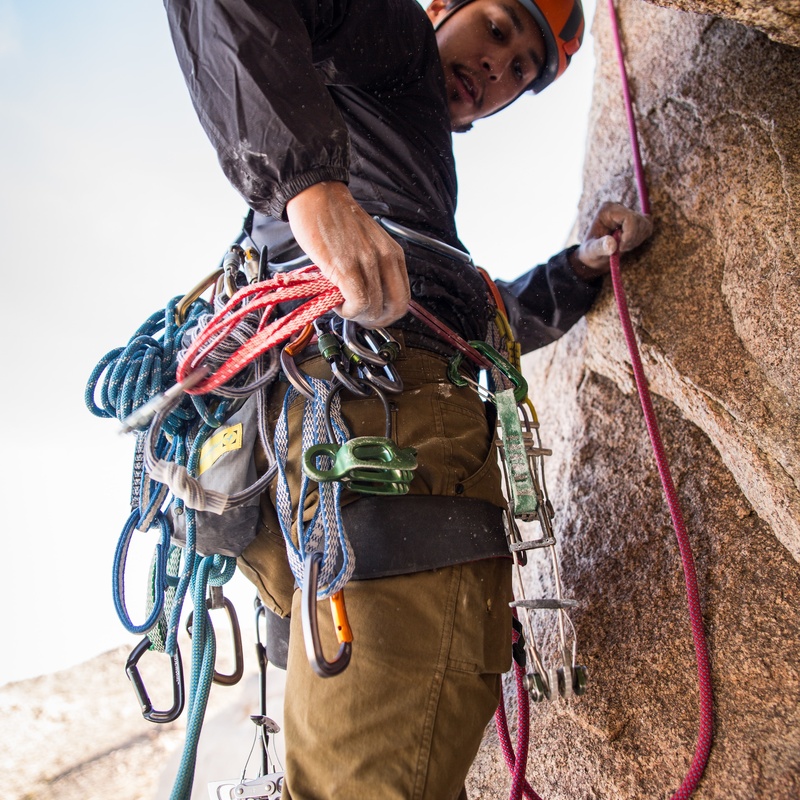
Gulp and commit hooks (gilp)
When creating a validation pipeline for your staged files, you don't have too many choices ending up with a huge bash script with countless functions for every linter, rule, or standard you want to automate.
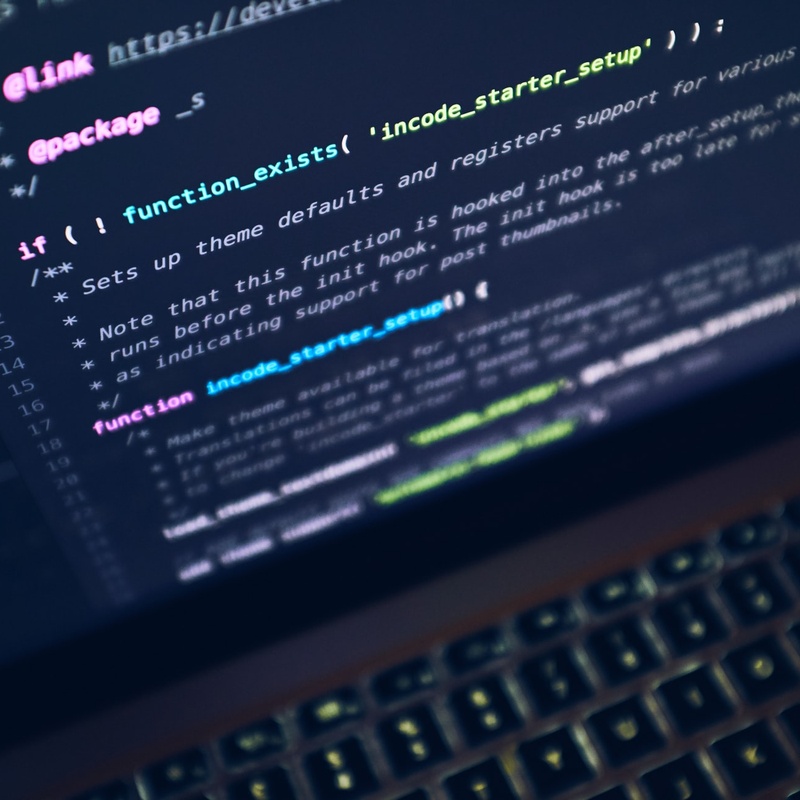
Checking commit messages and branch names (gilp-util)
This is part of Code quality enforcement series, we will investigate the utilities inside gilp-util for several use cases in the context of creating gulp plugins relying on git.
Photo by Cristopher Gower.
Categorized under nodejs / git / open source.We are Sophilabs
A software design and development agency that helps companies build and grow products by delivering high-quality software through agile practices and perfectionist teams.