The Power of Slice Notation in Python
Cesar Diaz
April 17, 2020
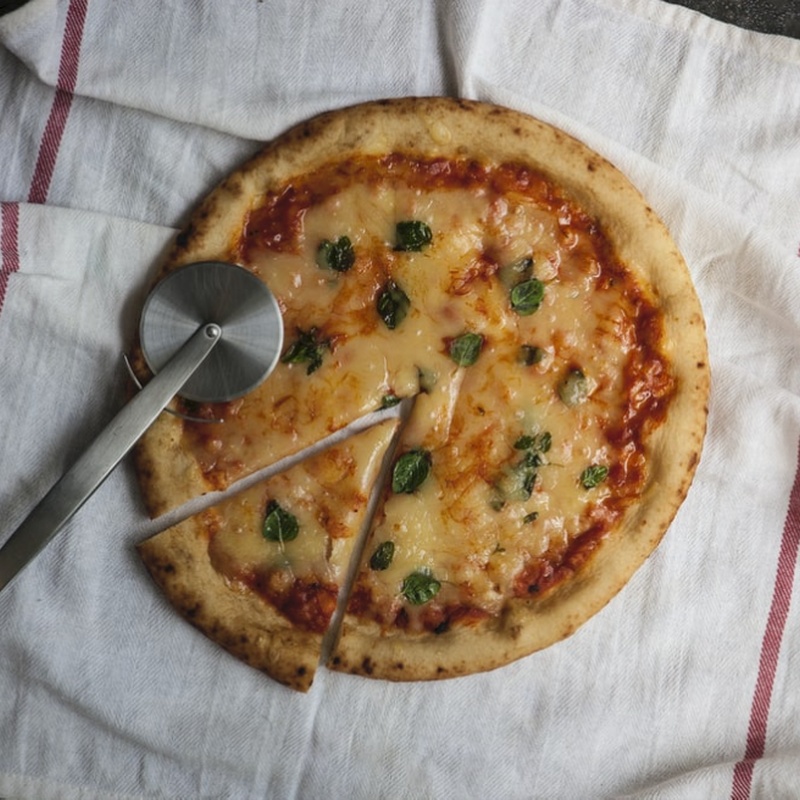
At Sophilabs, we have a lot of experience working with Python and Django. Today we will look more in-depth at the versatility of slice notation. It’s an important feature that can help you to achieve speed and readability in your code, as we’ll demonstrate below.
Let’s get started...
What Does Slice Notation Do?
Slice notation is a built-in feature of Python that allows access to parts of any string, list, or tuple. You can even modify or delete the items of mutable sequences, such as lists.
For example, let’s see how this works with a list called my_list
:
# [0,1,2,3,4,5,6,7,8,9]
my_list = range(10)
# would make a copy of all the list [0,1,2,3,4,5,6,7,8,9]
my_list[:]
# a copy from the start position through the rest of the list
my_list[start:]
# eg. my_list[1:] => [1,2,3,4,5,6,7,8,9]
# a copy from the beginning through stop -1
my_list[:stop]
# eg. my_list[:1] => [0]
# a copy from the start position through stop -1
my_list[start:stop]
# eg. my_list[1:8] => [1,2,3,4,5,6,7]
The full syntax includes a step option eg. my_list[start:stop:step]
. You can use this option if you want to skip values. By default, if the step is omitted in the declaration, the step value is 1.
This concept can go even further if the syntax uses extended notation:
# this will generate a new copy skipping the elements by the value of the step
my_list[::step]
# eg. my_list[::2] => [0,2,4,6,8]
Using Negative Values
One of the great things about Python is that you can use negative values as indexes, and of course slice notation is no exception.
Let’s return to our last example:
# will return the last element of the list
my_list[-value]
# eg. my_list[-2] => 8
# The last start elements of the list
my_list[-start:]
# eg. my_list[-2:] => [8, 9]
# will return everything except last stop elements of the list
my_list[:-stop]
# eg. my_list[:-2] => [0,1,2,3,4,5,6,7]
Similarly, with the extended version using steps, you can return a new list in reverse order using negative values in the step option:
my_list[::-step]
# eg. my_list[::-1] => [9,8,7,6,5,4,3,2,1,0]
# eg. my_list[::-2] => [9,7,5,3,1]
This means that we can make combinations that provide great flexibility, altering the order of the list. Furthermore, we can easily generate new structures using slice notation, making the code more versatile and readable.
Relationship with the Slice() Object
my_list[start:stop:step] is equivalent to my_list[slice(start, stop, step)]
To skip any argument, slice() must be replaced by None. For example:
# eg. my_list[::-2] => my_list[slice(None, None, -2)]
In a nutshell, we have applied slice notation in several ways, and as you can see, it is an extremely powerful tool. We explored some of the basics and this just the tip of the iceberg– use it in your projects and see the full potential of this feature.
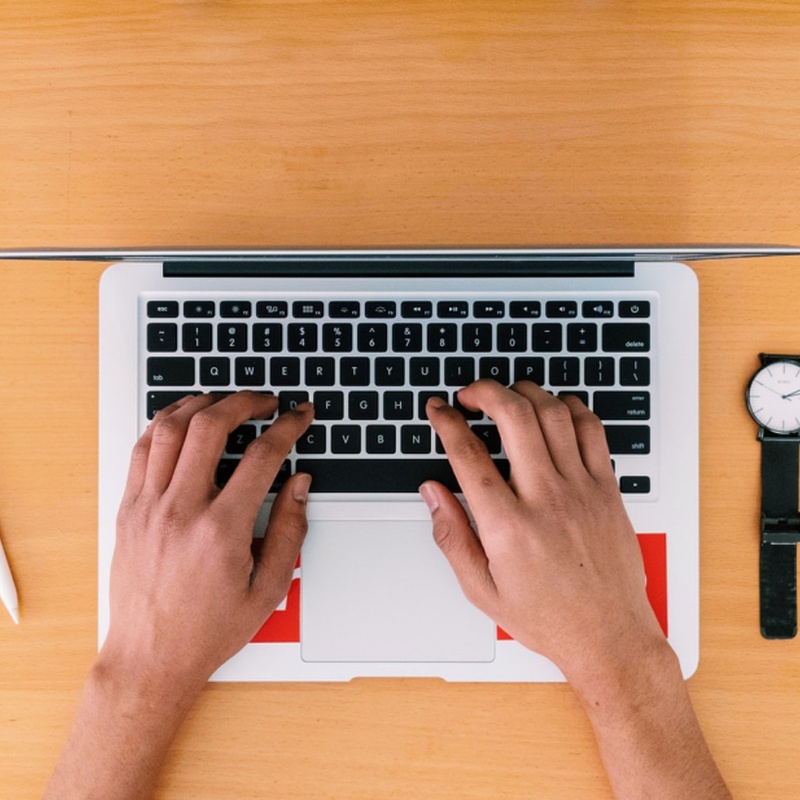
Complex Outer Joins with Django’s FilteredRelations
Today we will look at a problem that is trivial to solve with plain SQL but, until recently, couldn't be solved with the ORM without resorting to more complex approaches.
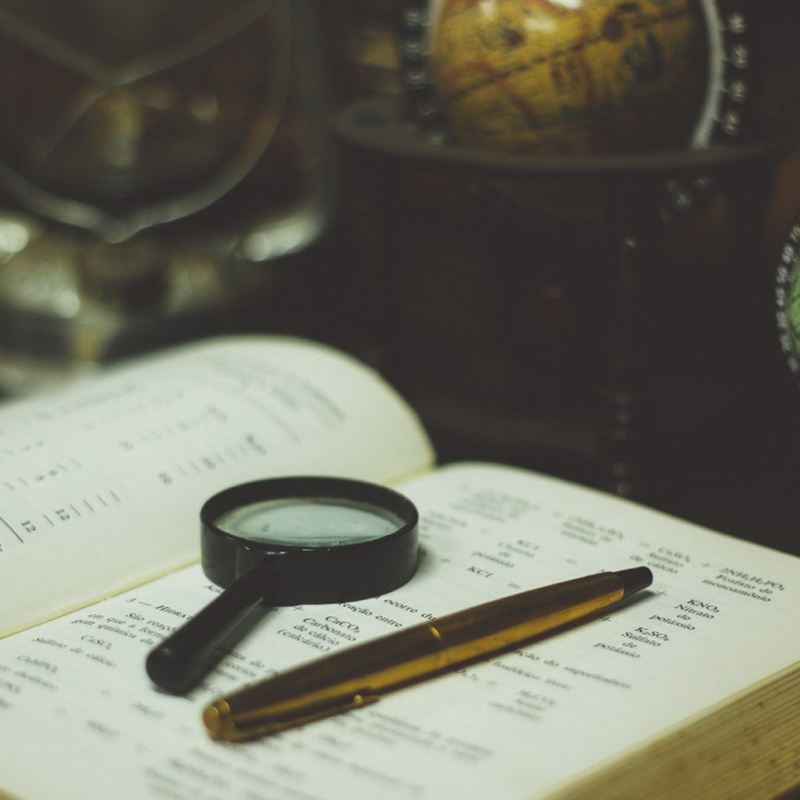
Why You Should Choose Your DBMS's Full Text Search Over a Dedicated Search Engine
If your project already uses a DBMS and you are uncertain whether to use a dedicated search engine, today we will share 3 reasons why you should *not*.
Photo by Heather Gill.
Categorized under research & learning.We are Sophilabs
A software design and development agency that helps companies build and grow products by delivering high-quality software through agile practices and perfectionist teams.